A couple of days ago I helped some developers put together a very basic web page that uses KeymanWeb to showcase their keyboard layout. The idea is that a visitor to the page will immediately be able to try out their keyboard layout without any fiddling around.
Another requirement was that the page work well on desktop and mobile devices. This requires a few little tricks, mostly because KeymanWeb has some special requirements to work smoothly on mobiles and tablets.
I’ve presented snippets of the code, in the order they appear in the document.
And here are screenshots just for posterity.
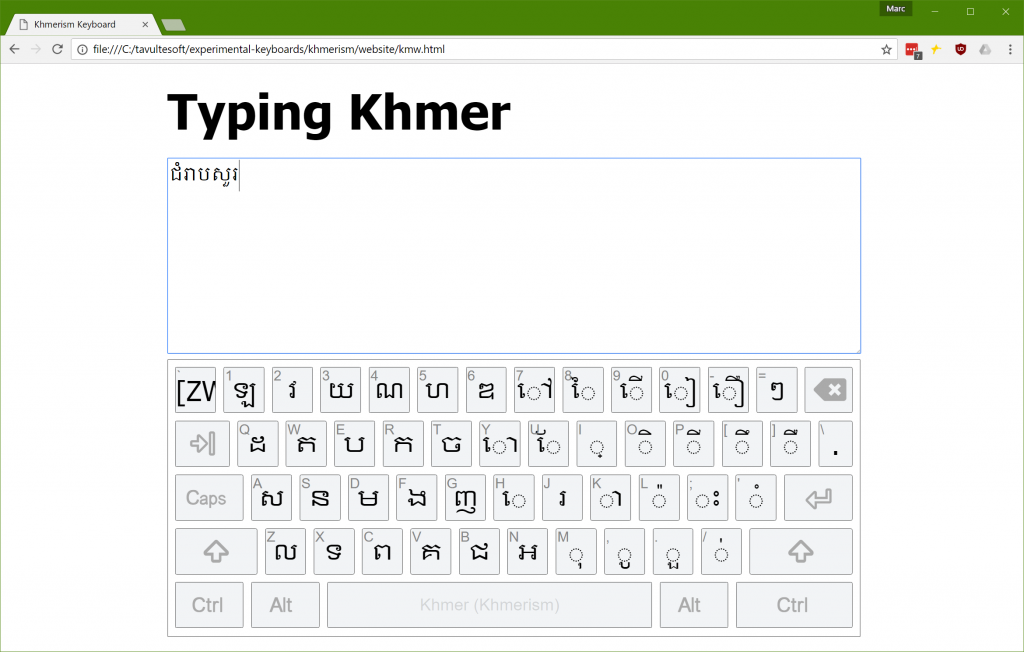
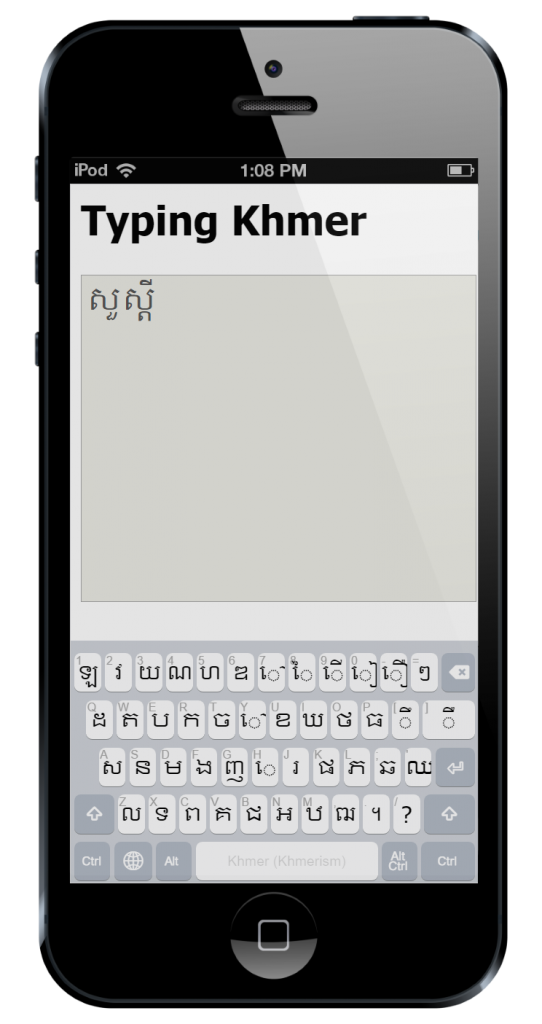
The usual HTML 5 boilerplate is thrown in at the top of the page. Our first trick is to put the page into an appropriate zoom and scroll mode for mobile and tablet devices with a viewport
meta tag. This is done with the following code:
<!-- at present, KMW requires this for mobile -->
<meta name="viewport" content="width=device-width, user-scalable=no">
At the time of writing, KeymanWeb (build 408) requires the viewport meta tag in order to render correctly on a variety of devices. Both clauses in the content attribute are required, and the keyboard will not scale correctly without them.
Next, I add a link to the stylesheet for the on screen keyboard. This is optional, because KeymanWeb will inject the link itself anyway, but it does prevent a Flash Of Unstyled Content.
<!-- this is optional but eliminates a flash of unstyled content -->
<link href='https://s.keyman.com/kmw/engine/408/src/osk/kmwosk.css' rel='stylesheet' type='text/css'>
I throw in some basic styling for the desktop and touch devices. You’ll see a selector of body.is-desktop
on a couple of the style selectors. I use this instead of a @media
query because this is differentiating between viewport-controlled devices and plain old boring desktop browsers. There may be a better way of doing this now, but I haven’t found it yet. The code for setting the is-desktop
class is found further on in this post.
/* Styles for the page */
* {
font-family: Tahoma, sans-serif;
}
body.is-desktop {
padding: 22px 2px 0 2px;
width: 900px;
margin: auto;
}
h1 {
font-size: 24pt;
font-weight: bold;
margin: 0 0 24px 0;
padding: 0;
}
body.is-desktop h1 {
font-size: 48pt;
}
/* Style the text area */
textarea {
width: 100%;
height: 250px;
font-family: "Khmer OS";
font-size: 24px;
}
I need to talk a bit more about the On Screen Keyboard (OSK) styling. While I could use the default styling (as shown below), this looks a little dated and I wanted to show how the keyboard could be styled as you like. There is some complexity to the OSK styling as it has many moving parts, around sizing, scaling and positioning of each individual key. I don’t want to throw away that baby, so instead I keep all the bathwater and just add some bubbles to jazz up the display the way I want it. That’s a mixed metaphor, but it was late when I wrote this.
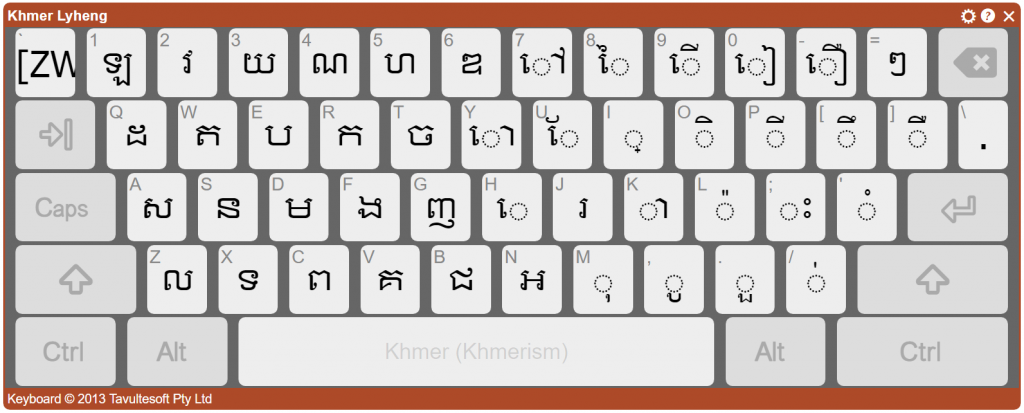
First, this CSS rule-set removes the thick red border and dark background, and adjusts the padding around the sides to compensate.
/* Style the on screen keyboard */
body .desktop .kmw-osk-inner-frame {
background: white;
border: solid 1px #404040;
padding-bottom: 2px;
padding-top: 8px;
}
Next, I hide the header and footer for the OSK. I don’t need them for this demo.
body .desktop .kmw-title-bar,
body .desktop .kmw-footer {
display: none;
}
I tweak the spacing between keys and rows and make the keys slightly less rounded.
body .desktop .kmw-key-square {
border-radius: 2px;
padding-bottom: 8px;
}
body .desktop .kmw-key {
border-radius: 2px;
border: solid 1px #404040;
background: #f2f4f6;
}
body .desktop .kmw-key:hover {
background: #c0c8cf;
}
And that’s it for the CSS changes. It’s really pretty easy to restyle the keyboard without losing the benefits of a scalable, cross-platform keyboard. You’ll note that sneaky .desktop selector creeping in there. That’s because I’ve opted to style only the desktop keyboard; the touch layouts are already pretty nice and I’ll keep them as is.
I load the KeymanWeb code from the KeymanWeb CDN. You can load from the KeymanWeb CDN (running on Azure) or keep a locally hosted copy, of course. I’ve commented out the source version and because we have only a single keyboard, I have elected not to include a menu for switching languages.
<!-- Uncomment these lines if you want the source version of KeymanWeb (and remove the keymanweb.js reference)
<script src="https://s.keyman.com/kmw/engine/408/src/kmwstring.js"></script>
<script src="https://s.keyman.com/kmw/engine/408/src/kmwbase.js"></script>
<script src="https://s.keyman.com/kmw/engine/408/src/keymanweb.js"></script>
<script src="https://s.keyman.com/kmw/engine/408/src/kmwosk.js"></script>
<script src="https://s.keyman.com/kmw/engine/408/src/kmwnative.js"></script>
<script src="https://s.keyman.com/kmw/engine/408/src/kmwcallback.js"></script>
<script src="https://s.keyman.com/kmw/engine/408/src/kmwkeymaps.js"></script>
<script src="https://s.keyman.com/kmw/engine/408/src/kmwlayout.js"></script>
<script src="https://s.keyman.com/kmw/engine/408/src/kmwinit.js"></script>
-->
<script src="https://s.keyman.com/kmw/engine/408/keymanweb.js"></script>
<!-- Uncomment if you want the user to be able to switch keyboards with a toggle popup ...
or you can create a custom switch if you prefer
<script src='https://s.keyman.com/kmw/engine/408/kmwuitoggle.js'></script>
-->
Now, no self-respecting web developer is going to include inline script, except in a Hello World demo. So let’s call this the KeymanWeb Hello World demo, so I can keep my self respect. Or something.
I use a simple page load listener. You could use an earlier listener, such as DOMContentLoaded
, but window.onload
has a rich and nearly honourable history.
// This should really be in a separate file.
// But for now in one file is easier to understand
// After everything has loaded
window.addEventListener('load', function() {
Next some voodoo. Allows me to style the touch and desktop versions differently, as I touched on earlier. Why? Because KeymanWeb works very differently on touch devices. If you think about this, it must be so. A touch device has its own soft keyboard, which KeymanWeb must, by slightly convoluted means, hide, and replace with its own soft keyboard. Whereas, on a desktop device, KeymanWeb can show a utility soft keyboard, but does most of its interaction by getting in there between the hardware keyboard and the input fields.
Don’t ask me about Windows touch devices. Does anyone actually use those? (Okay, I’m sure they do, but it’s a rocky path for us poor keyboard devs to tread!)
if(!tavultesoft.keymanweb.util.isTouchDevice()) {
document.body.className += 'is-desktop';
}
Next, make sure KeymanWeb is initialised. If I don’t do this myself, KeymanWeb will do so when things are ready, but I need KeymanWeb to be ready in order to load keyboards and attach events, and so on.
tavultesoft.keymanweb.init();
I could add another keyboard, or a stock keyboard from the repository. I haven’t, but this shows you how.
//tavultesoft.keymanweb.addKeyboards('@eng'); // Loads default English keyboard from Keyman Cloud (CDN) if you want it
In this case, I have a custom keyboard, developed by Lyheng Phuoy. This is an early beta version of the keyboard but already it’s very impressive.
// Add our custom keyboard
tavultesoft.keymanweb.addKeyboards({
name: 'Khmerism', // Display name of the keyboard
id: 'lyhengkeyboard', // ID of the keyboard for reference in code
filename: './lyhengkeyboard-1.0.js', // source of the keyboard for dynamic load
version: '1.0', // version of the keyboard, optional
language: [{
name: 'Khmer', // language name for UI elements
id: 'khm', // language ID for tagging text
region: 'as' // region of the language, for UI elements, optional
}]
});
In this section, I want to control the size, position and flexibility of the keyboard. I don’t want it to be resizable or movable. So I set nomove
and nosize
accordingly.
var ta = document.getElementsByTagName('textarea')[0];
// Watch for the keyboard being shown and set its position
tavultesoft.keymanweb.osk.addEventListener('show', function() {
var rectParams = {
left: ta.offsetLeft,
top: ta.offsetTop + ta.offsetHeight + 8, // a small gap below the text area
width: ta.offsetWidth,
height: ta.offsetWidth * 0.4, // a pleasing aspect ratio
nomove: true,
nosize: true
};
tavultesoft.keymanweb.osk.setRect(rectParams);
});
// Focus text area after everything loads
ta.focus();
Finally, in the body element, I set a special magic class, osk-always-visible
, so that KeymanWeb doesn’t hide its on screen keyboard after displaying it the first time. And then we have basically the world’s simplest page with a textarea
.
<!-- The osk-always-visible class tells KeymanWeb not to hide the osk on blur, after it is
shown the first time -->
<body class="osk-always-visible">
<h1>Typing Khmer</h1>
<textarea rows="6" cols="60"></textarea>
</body>
And that’s it! I’d love to see what you do with KeymanWeb on your own sites.
Here’s the full keyboard source, and that link to the demo page again, including Lyheng’s keyboard (with his permission).