Update: Photos now included. All photos bar the last one courtesy of Rob Cumine.
There was quite a bit of buzz leading up to the initial stage of the 2011 Tour of Tasmania, an 18km team time trial (TTT) up the 1200m Mt Wellington climb starting from Cascade Brewery. As various commentators described the stage as “innovative”, “unique”, “ground-breaking”, and even “stupid”, I was keen to see for myself how it would pan out. I was a little dubious as to how it would work, but it turned out to be a spectacular way to shake up the General Classification and inject some spice and suspense early in the race.
So why the controversy? While up-hill individual time trials are common, the tour organisers claimed that this was a world-first for the team time trial format. Some riders suggested that only a team stacked with climbers could win, and this meant it was not a sensible format. But I wondered if that was any different to having a team stacked with time trial specialists for a traditional TTT.
I took the day off work to watch the stage. The day dawned foggy and pretty chilly; together with my mates Ant and Phil, we scouted out the climb early in the morning, then descended back to the start line to meet the rest of the group who would join us to cheer on the pros. The mid-morning descent left all of us shivering and I found myself in that zone where one struggles to care enough to keep focused on the road. Thick fog on the descent kept our speed at about 20km/h, cars looming out of the mist at the last moment to keep us on our toes. Even at that low speed, we still froze. Fortunately, the fog thinned out a bit by the time the race started, but half the climb was still fog shrouded.
After joining the rest of the group at Cascade Brewery, we climbed at a much more leisurely pace for a second time, before selecting a vantage point about 2km from the summit to watch the race unfolding. Our vantage point was chosen mostly on the basis of it being a bit sunny!
The race favourites were definitely Genesys Wealth Advisors, given that they’ve won every National Road Series race this year so far, as well as collecting a hefty swag of stage wins. But they expected a challenge from the Russian National Team and Jayco/2XU, and a challenge they got! In this TTT there was not a time cut, and the top 4 riders for each team all got the time of the 4th rider over the line. For those teams with 4 riders, it was just pure survival. Larger teams could afford to burn up some domestiques in the faster first third of the climb up Strickland Ave.
I think the key image I took away was the pain etched into so many riders’ faces, especially the 4th rider of most teams — I’m sure this would have been a resounding personal best time for many of these riders, while the strongest climbers on each team had it relatively easier. In a typical TTT, stronger time triallers do take longer stints on the front, but in this climb I’m guessing the stronger climbers were on the front the whole way to the top!
Most teams were down to four riders as they passed us, and in a couple of cases the fourth rider was really struggling, with lots of urging and encouragement from team members and director in the team car following.
Jayco/2XU gave us a new call for future group rides. No more “easy on the front” calls: as they rode past us, the leading rider accelerated slightly, provoking a heartrending cry from the fourth rider, “what on earth are you doing?!?” and the team director panicking a little with a “hey hey hey hey hey” yelled out of the car window. Even in 2-3 seconds that little acceleration had opened up a sizeable gap in the group.
Also amusing was local rider Danny Pulbrook of Pure Tasmania tonking past on a green mountain bike borrowed from Scott of Ray Appleby Cycles after apparently destroying his derailleur earlier in the climb. He nearly stopped to swap to one of our road bikes but decided he couldn’t afford to stop again…
After the last Genesys rider passed, we hopped back on our bikes and followed him to the summit. By that point I had put on my winter rain jacket as I was getting pretty cold, so I was surprised by how many riders opted to ride down given the chilly, foggy descent!
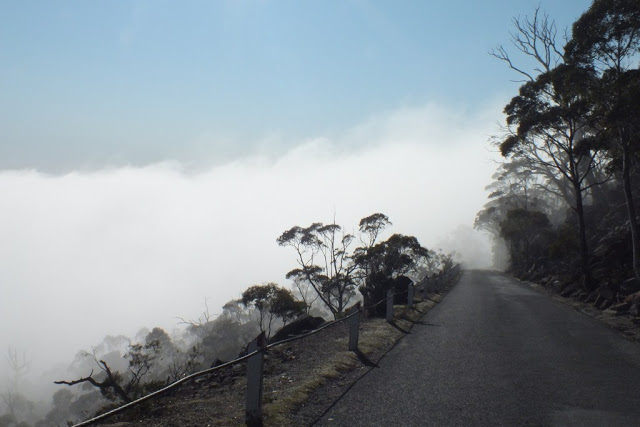 |
Heading down afterwards, approaching the fog (R.Cumine) |
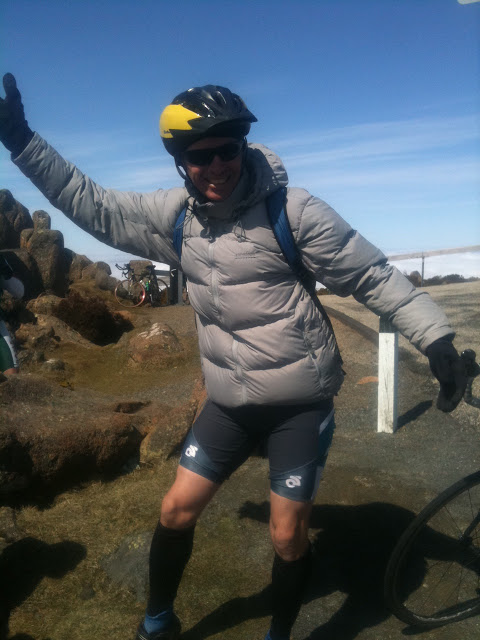 |
So he’s not one of the pros, but at least he’ll survive the descent, right? |
So what’s the verdict? Jayco/2XU took line honours with a strong group of climbers; Genesys managed 2nd place, 17 seconds behind the leaders, with the Russians a further 17 seconds back. The results were closer than I expected, with all teams finishing within 8 minutes of the leading team, and the last rider crossing the line 20 minutes behind the leader. The stage essentially became a test of each team’s ability to encourage its riders to climb faster, but it was much more dependent on having 4 strong climbers than a traditional TTT where stronger riders do more work — no chance of any real recovery behind the other riders on a climb! The format actually worked a lot better than I expected, and kudos to the tour directors for generating some press and interest in the race this way. That said, however, I don’t reckon we’ll be seeing a TTT hill climb in the major international tours any time soon.
Now forget about the pros; what about our times? I came in at 1:09:24, about 1 minute behind the slowest “pro”; Ant is a great climber, and was 3.5 minutes ahead of me (and looked fresh as a daisy at the summit), and Phil 2 minutes ahead of me — and they waited for me at one point. But at least we did the mountain twice!